現在お買い物カゴには何も入っていません。
コンテンツ制作支援プログラム
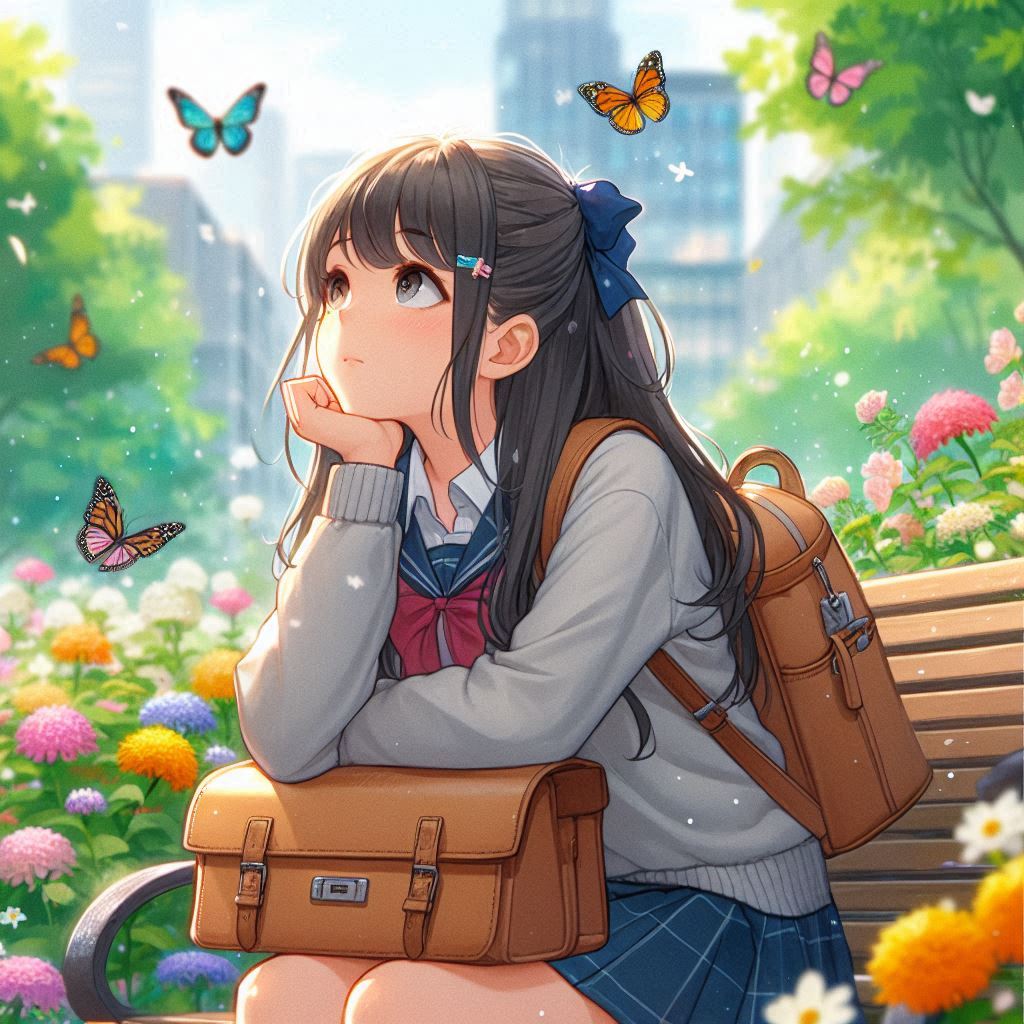
AIとPythonを利用してスプレッドシートなどのコンテンツのトレンド分析、ネタのランキング、そして脚本をスプレッドシートやドキュメントに出力するコードですね。以下に、その実現方法と具体的なコード例を示します。
1. 全体的な構成
このシステムは、大きく以下の3つの部分から構成されます。
- トレンド分析 & ネタランキング (Python)
- Google Trends API, Twitter API, YouTube Data API などを使って、キーワードの検索ボリュームや関連トピック、急上昇ワードなどを取得します。
- 取得したデータを分析し、トレンドのキーワードやトピックを抽出します。
- ネタのランキングをスコアリングし、上位のネタを選定します。
- 脚本生成 (Python / AI)
- OpenAIのGPT-3やGPT-4などの大規模言語モデル (LLM) を利用し、ランキング上位のネタを基に脚本を生成します。
- プロンプトエンジニアリングにより、脚本のスタイル(コメディ、ドラマ、ホラーなど)、登場人物、大まかなプロットなどを指定します。
- 生成された脚本を整形します。
- 出力 (Python)
- 生成された脚本を、Google スプレッドシートや Google ドキュメントに出力します。
- トレンド分析の結果やネタランキングも、必要に応じてスプレッドシートに出力します。
2. 必要なライブラリ/API
- トレンド分析 & ネタランキング
pytrends
(Google Trends API)tweepy
(Twitter API)google-api-python-client
(YouTube Data API, Google Sheets API, Google Docs API)requests
(汎用的なHTTPリクエスト)beautifulsoup4
(HTML解析)pandas
(データ分析)numpy
(数値計算)
- 脚本生成
openai
(OpenAI API)
- 出力
gspread
(Google Sheets API のラッパー)google-auth
(Google API 認証)
3. コード例 (簡略版)
以下は、各部分のコード例を簡略化したものです。実際には、APIキーの取得、エラーハンドリング、詳細なデータ処理などが必要になります。
Python
import pytrends
from pytrends.request import TrendReq
import tweepy
import openai
import gspread
from google.oauth2.service_account import Credentials
import pandas as pd
import datetime
from datetime import date, timedelta
# --- (1) トレンド分析 & ネタランキング ---
# Google Trends
def get_google_trends(keywords, timeframe='today 3-m'):
pytrends = TrendReq(hl='ja-JP', tz=360) # 日本語、タイムゾーン設定
pytrends.build_payload(keywords, cat=0, timeframe=timeframe, geo='JP', gprop='')
return pytrends.interest_over_time()
# Twitter (要APIキー設定)
def get_twitter_trends(consumer_key, consumer_secret, access_token, access_token_secret):
# Tweepy OAuth handler object
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth)
# WOEID of Japan
trends = api.get_place_trends(id=23424856)
return [trend['name'] for trend in trends[0]['trends']]
# 例: Google Trends と Twitter Trends を組み合わせてキーワードをスコアリング
def rank_keywords(google_keywords, twitter_keywords):
google_trends_df = get_google_trends(google_keywords)
# スコア計算 (例: Google Trends の検索ボリュームと、Twitterでの言及数を組み合わせる)
keyword_scores = {}
for keyword in google_keywords:
# 過去3ヶ月での最大検索ボリュームを合計
try:
keyword_scores[keyword] = google_trends_df[keyword].max()
except KeyError: # キーワードが存在しない場合
keyword_scores[keyword] = 0
#twitterでトレンド入りしているワードはスコアを加算する
for tw_keyword in twitter_keywords:
for g_keyword in google_keywords:
if tw_keyword in g_keyword:
keyword_scores[g_keyword] += 10 #Twitterトレンドの重みづけは要調整
# スコアで降順ソート
ranked_keywords = sorted(keyword_scores.items(), key=lambda x: x[1], reverse=True)
return ranked_keywords
# --- (2) 脚本生成 (OpenAI API) ---
def generate_script(prompt, openai_api_key):
openai.api_key = openai_api_key
response = openai.Completion.create(
engine="text-davinci-003", # または、より高性能なモデル (例: gpt-4)
prompt=prompt,
max_tokens=1500, # 生成するトークン数の上限
n=1, # 生成する脚本の数
stop=None, # 停止トークン (例: "\n\n")
temperature=0.7, # 生成の多様性 (0.0 ~ 1.0)
)
return response.choices[0].text.strip()
# --- (3) 出力 (Google スプレッドシート) ---
def output_to_spreadsheet(data, spreadsheet_id, credentials_path, sheet_name="Sheet1"):
# 認証情報
scopes = [
'https://www.googleapis.com/auth/spreadsheets',
'https://www.googleapis.com/auth/drive'
]
credentials = Credentials.from_service_account_file(credentials_path, scopes=scopes)
gc = gspread.service_account(filename=credentials_path)
# スプレッドシートを開く
sh = gc.open_by_key(spreadsheet_id)
worksheet = sh.worksheet(sheet_name)
#既存のシートのデータ数を取得
existing_data = worksheet.get_all_values()
start_row = len(existing_data) + 1
# データを出力 (例: リストをそのまま出力)
if isinstance(data, list):
if isinstance(data[0], tuple): # (キーワード, スコア) のタプルのリスト
# 日付をヘッダーに追加
today = date.today()
formatted_date = today.strftime('%Y-%m-%d')
worksheet.update_cell(start_row, 1, formatted_date)
start_row += 1
worksheet.append_row(["Rank", "Keyword", "Score"]) # ヘッダー行
for i, (keyword, score) in enumerate(data):
worksheet.append_row([i+1, keyword, score])
elif isinstance(data[0], list):#二次元配列
worksheet.update('A' + str(start_row) ,data)
else: #一次元配列
worksheet.update('A'+ str(start_row), [[item] for item in data])
elif isinstance(data, str):#文字列
# 既存のコンテンツを取得
existing_content = worksheet.get('A1')
if existing_content:
updated_content = existing_content[0][0] + "\n\n" + data if existing_content[0][0] else data
else:
updated_content = data
# セルA1に追記
worksheet.update_cell(1, 1, updated_content)
else:
print("Error: Unsupported data type for spreadsheet output.")
# --- メイン処理 (例) ---
if __name__ == "__main__":
# --- 1. トレンド分析 ---
# Google Trends 用のキーワードリスト
google_keywords = ["AI", "メタバース", "ブロックチェーン", "SDGs","ChatGPT"]
# Twitter API のキー (各自で取得・設定)
consumer_key = "YOUR_CONSUMER_KEY"
consumer_secret = "YOUR_CONSUMER_SECRET"
access_token = "YOUR_ACCESS_TOKEN"
access_token_secret = "YOUR_ACCESS_TOKEN_SECRET"
twitter_trends = get_twitter_trends(consumer_key, consumer_secret, access_token, access_token_secret)
# キーワードランキング
ranked_keywords = rank_keywords(google_keywords, twitter_trends)
print("Ranked Keywords:", ranked_keywords)
# --- 2.脚本生成 ---
# OpenAI APIキー (各自で取得・設定)
openai_api_key = "YOUR_OPENAI_API_KEY"
# 脚本生成のプロンプト (ランキング上位のキーワードを使用)
top_keyword = ranked_keywords[0][0] # 最もランキングの高いキーワード
prompt = f"""
以下の情報を元に、日本語でコントの脚本を作成してください。
* テーマ: {top_keyword}
* 登場人物: 2人 (AとB)
* 設定: 未来のカフェ
* スタイル: 面白おかしく、{top_keyword}の現状と課題を風刺する
脚本:
"""
script = generate_script(prompt, openai_api_key)
print("Generated Script:\n", script)
# --- 3. スプレッドシート出力 ---
spreadsheet_id = "YOUR_SPREADSHEET_ID" # スプレッドシートID
credentials_path = "path/to/your/credentials.json" # 認証情報JSONファイルへのパス
# ランキング結果をスプレッドシートに出力 (シート名: Trend Ranking)
output_to_spreadsheet(ranked_keywords, spreadsheet_id, credentials_path, sheet_name="Trend_Ranking")
# 生成された脚本をスプレッドシートに出力 (シート名: Scripts, 既存の内容に追記)
output_to_spreadsheet(script, spreadsheet_id, credentials_path, sheet_name="Scripts")
#twitterトレンドをスプレッドシートに出力(シート名: Twitter Trends, 既存の内容に追記)
output_to_spreadsheet(twitter_trends, spreadsheet_id, credentials_path, sheet_name="Twitter_Trends")
4. 重要なポイントと改善案
- APIキーの管理: APIキーは、コード内に直接書き込まず、環境変数や設定ファイルから読み込むようにしましょう。
- エラーハンドリング: APIリクエストの失敗、データの欠損、予期せぬ形式のデータなど、さまざまなエラーに対処するコードが必要です。
- データ処理の効率化: 大量のデータを扱う場合は、
pandas
やnumpy
の機能を活用して処理を効率化しましょう。 - プロンプトエンジニアリング: LLMから高品質な脚本を生成するためには、プロンプトの設計が非常に重要です。様々なプロンプトを試して、最適なものを見つけましょう。
- モデルの選択: OpenAI APIのモデルは、
text-davinci-003
以外にも、gpt-3.5-turbo
(チャット形式に最適化) やgpt-4
(より高性能) などがあります。目的に応じて使い分けましょう。 - Google ドキュメントへの出力: Google Docs API を使えば、スプレッドシートだけでなく、ドキュメントにも直接出力できます。書式設定なども可能です。
- 定期実行: このシステムを定期的に実行 (例: 毎日、毎週) することで、常に最新のトレンドに基づいたコンテンツ生成が可能になります。cron (Linux) や タスクスケジューラ (Windows) を利用しましょう。
- 著作権、利用規約の遵守: 各種APIの利用規約、生成されたコンテンツの著作権に十分注意してください。特に商用利用の場合は注意が必要です。
このコードと解説が、あなたのコンテンツ制作の自動化に役立つことを願っています。
投稿者:
タグ:
コメントを残す